How To Upload Files From Github To Smarterasp
If you lot demand to upload a file to GitHub, you can exercise it easily from github.com. However, that doesn't scale. When it comes to doing information technology on a repeated footing or facilitating automation, yous'll want to take a programmatic approach. While GitHub does have a Residue API, C# developers can take reward of the Octokit library. This library saves you lot a lot of fourth dimension—you can take advantage of dynamic typing, get data models automatically, and non have to construct HttpClient
calls yourself.
This postal service volition show you how to utilise the Octokit library to upload a Markdown file to a GitHub repository.
Before we get started, download the Octokit library from NuGet. You can do this in several different ways, but the simplest is using the dotnet
CLI. From the path of your project, execute the following from your favorite concluding:
dotnet add parcel Octokit
Create the client
To become started, you lot'll need to create a customer and then that Octokit can connect to GitHub. To do that, you can instantiate a new GitHubClient
. The GitHubClient
takes a ProductHeaderValue
, which tin can be whatsoever cord—information technology's so GitHub tin can place your application. GitHub doesn't permit API requests without a User-Agent
header, and Octokit uses this value to populate 1 for yous.
//using Octokit; var gitHubClient = new GitHubClient(new ProductHeaderValue("MyCoolApp"));
With this unmarried line of code, yous can now access public GitHub information. For case, you can utilise a web browser to get a user'southward details. Here's what you get for api.github.com/users/daveabrock
:
{ "login": "daveabrock", "id": 275862, "node_id": "MDQ6VXNlcjI3NTg2Mg==", "avatar_url": "https://avatars.githubusercontent.com/u/275862?v=4", "gravatar_id": "", "url": "https://api.github.com/users/daveabrock", "html_url": "https://github.com/daveabrock", "followers_url": "https://api.github.com/users/daveabrock/followers", "following_url": "https://api.github.com/users/daveabrock/following{/other_user}", "gists_url": "https://api.github.com/users/daveabrock/gists{/gist_id}", "starred_url": "https://api.github.com/users/daveabrock/starred{/possessor}{/repo}", "subscriptions_url": "https://api.github.com/users/daveabrock/subscriptions", "organizations_url": "https://api.github.com/users/daveabrock/orgs", "repos_url": "https://api.github.com/users/daveabrock/repos", "events_url": "https://api.github.com/users/daveabrock/events{/privacy}", "received_events_url": "https://api.github.com/users/daveabrock/received_events", "type": "User", "site_admin": simulated, "name": "Dave Brock", "company": null, "web log": "daveabrock.com", "location": "Madison, WI", "email": cypher, "hireable": null, "bio": "Software engineer, Microsoft MVP, speaker, blogger", "twitter_username": "daveabrock", "public_repos": 55, "public_gists": 2, "followers": 63, "following": 12, "created_at": "2010-05-13T20:05:05Z", "updated_at": "2021-03-13T19:05:32Z" }
(Fun fact: it'southward fun to wait at the id
values to see how long a user has been with GitHub—I was the 275,862nd registered user, a few years later GitHub co-founder Tom Preston-Warner, who has an id
of 1
.)
To go this data programmatically, yous tin use the User
object:
//using Octokit; var gitHubClient = new GitHubClient(new ProductHeaderValue("MyCoolApp")); var user = wait gitHubClient.User.Get("daveabrock"); Panel.WriteLine($"Woah! Dave has {user.PublicRepos} public repositories.");
That was quick and piece of cake, only non very much fun. To modify annihilation in a repository y'all'll need to authenticate.
Authenticate to the API
You tin authenticate to the API using 1 of ii means: a basic username/password pair or an OAuth flow. Using OAuth (from a generated personal access token) is well-nigh always a better approach, as you won't have to store a password in the code, and you tin can revoke it as needed. What happens when a countersign changes? Bad, bad, bad.
Instead, connect past passing in a personal access token (PAT). From your profile details, navigate to Developer settings > Personal admission tokens, and create one. You'll desire to include the repo
permissions. Later on you create it, copy and paste the token somewhere. Nosotros'll demand information technology shortly.
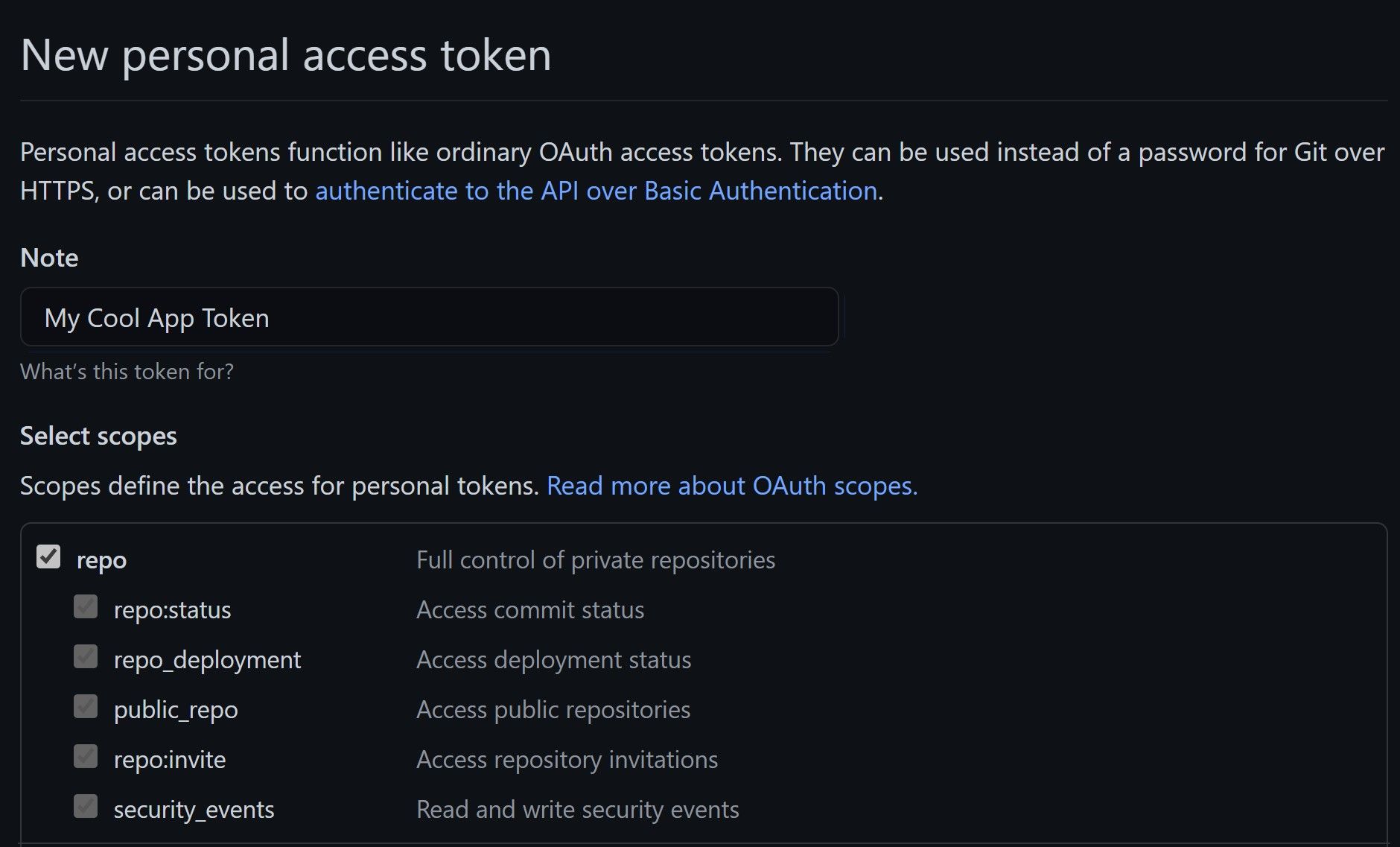
With the generated PAT, here'south how you lot cosign to the API. (Note: Be careful with your token. In real-world scenarios, brand sure to store information technology somewhere safe and access it from your configuration.)
//using Octokit; var gitHubClient = new GitHubClient(new ProductHeaderValue("MyCoolApp")); gitHubClient.Credentials = new Credentials("my-new-personal-access-token");
Add a new file to the repository
With that in identify, I'chiliad using a StringBuilder
to create a elementary Markdown file. Here's what I have so far:
//using Octokit; var gitHubClient = new GitHubClient(new ProductHeaderValue("MyCoolApp")); gitHubClient.Credentials = new Credentials("my-new-personal-admission-token"); var sb = new StringBuilder("---"); sb.AppendLine(); sb.AppendLine($"date: \"2021-05-01\""); sb.AppendLine($"title: \"My new fancy postal service\""); sb.AppendLine("tags: [csharp, azure, dotnet]"); sb.AppendLine("---"); sb.AppendLine(); sb.AppendLine("# The heading for my first mail"); sb.AppendLine();
Because I need to pass in a cord to create my file, I'll utilise sb.ToString()
, to pass it in. I can call the CreateFile
method to upload a file now.
//using Octokit; var gitHubClient = new GitHubClient(new ProductHeaderValue("MyCoolApp")); gitHubClient.Credentials = new Credentials("my-new-personal-access-token"); var sb = new StringBuilder("---"); sb.AppendLine(); sb.AppendLine($"date: \"2021-05-01\""); sb.AppendLine($"title: \"My new fancy updated post\""); sb.AppendLine("tags: [csharp, azure, dotnet]"); sb.AppendLine("---"); sb.AppendLine(); sb.AppendLine("The heading for my first post"); sb.AppendLine(); var (owner, repoName, filePath, branch) = ("daveabrock", "daveabrock.github.io", "_posts/2021-05-02-my-new-mail service.markdown", "chief"); look gitHubClient.Repository.Content.CreateFile( owner, repoName, filePath, new CreateFileRequest($"First commit for {filePath}", sb.ToString(), co-operative));
You lot can fire and forget in many cases, but it'due south proficient to note this method returns a Task<RepositoryChangeSet>
which gives you back commit and content details.
Update an existing file
If you try to execute CreateFile
on an existing file, you'll get an error that the SHA for the commit doesn't exist. You'll need to fetch the SHA from the result showtime.
var sha = result.Commit.Sha; await gitHubClient.Repository.Content.UpdateFile(owner, repoName, filePath, new UpdateFileRequest("My updated file", sb.ToString(), sha));
In many scenarios, yous won't exist editing the file right later you created it. In these cases, get the file details first:
var fileDetails = await gitHubClient.Repository.Content.GetAllContentsByRef(owner, repoName, filePath, co-operative); var updateResult = wait gitHubClient.Repository.Content.UpdateFile(owner, repoName, filePath, new UpdateFileRequest("My updated file", sb.ToString(), fileDetails.First().Sha));
What almost base64?
The CreateFile
call also has a convertContentToBase64
boolean flag, if you'd prefer. For example, I tin pass in an prototype'southward base64 cord and set convertContentToBase64
to truthful
.
string imagePath = @"C:\pics\headshot.jpg"; string base64String = GetImageBase64String(imagePath); var result = await gitHubClient.Repository.Content.CreateFile( possessor, repoName, filePath, new CreateFileRequest($"First commit for {filePath}", base64String, branch, true)); static cord GetImageBase64String(string imgPath) { byte[] imageBytes = System.IO.File.ReadAllBytes(imgPath); return Convert.ToBase64String(imageBytes); }
Wrap up
I showed yous how to apply the Octokit C# library to upload a new file to GitHub in this post. We also discussed how to update existing files and pass base64 strings to GitHub equally well. Thanks for reading, and have fun!
How To Upload Files From Github To Smarterasp,
Source: https://www.daveabrock.com/2021/03/14/upload-files-to-github-repository/
Posted by: murphydoculd.blogspot.com
0 Response to "How To Upload Files From Github To Smarterasp"
Post a Comment